Page Object Model is an object design pattern that is popularly used in test automation for a better testing experience. In this technique, a Page class is created for each web page of the application. This Page class contains web elements and methods for action to be performed on these web elements. These web elements and methods are accessed by test cases, which are written in a separate Test class file.
Consider that you need to perform login action before booking a flight and viewing the itinerary. The login function is common for both booking flights and viewing itinerary scenarios. Instead of writing login web elements and methods in each page class, we can create one login page class, and reuse it in other page classes.
Cypress, a popular automation framework, provides features to support Page Object Model. In this article, we will cover the setup and end-to-end flow for the Page Object Model using Cypress (version 10).
Cypress E2E Page Object Model
To demonstrate the working of the page object model in Cypress, we will use newtoursdemosite as a demo site. We will implement the 'Login' scenario in this example. Let's start!
Step 1
Create a 'pageobjects' folder under the 'cypress' folder of the project, which will store the page classes.
Step 2
Create a page class 'loginPage.js' under the 'pageobjects' folder. In 'loginPage.js', we will store the web elements and write methods to perform login functionality.
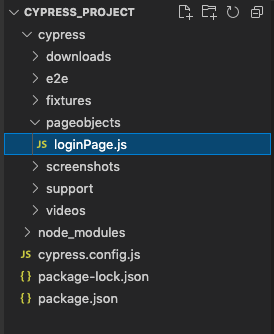
Step 3
In loginPage.js, create a 'loginPage' class that we will export to the test spec file using 'export'.
Inside the class, create an object named 'elements'. 'elements' object contains a set of key and value pairs for locating web elements on the login page.
Then write methods for the following actions:
- Enter username
- Enter password
- Click Submit
Step 4
Create a 'tests' folder under the 'e2e' folder, which will store all test cases. Now, create a test spec file 'verifyLogin.spec.cy.js' under the 'tests' folder.

Note: For the cypress version below 10, create a 'tests' folder under 'integration'.
Step 5
Import loginPage.js class to access its methods. Then create an instance of the loginPage.js class and call the respective methods.
Step 6
Run the test in Cypress
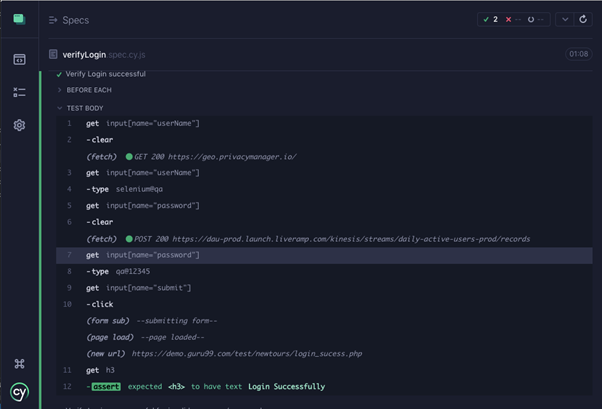
Using Getter and Setter
You need to create a "get" and "set" command to define Object accessors. The advantage of using getter and setter is that we can use them as properties instead of functions.
Without Getter
With Getter
loginObj.enterUsername('selenium@qa')
loginObj.username.type('selenium@qa')
loginPage.js class file
verifyPage.spec.cy.js class file
Test result

Note: Find the sample code snippets for these features in the GitHub repository here.
Conclusion
Go ahead and implement Page Object Model using Cypress in your project. And improve test readability, and code maintainability, and reduce code duplication.
Happy Testing!