Opening an URL in a new window or new tab is one of the features provided by Selenium 4. In Selenium 3, we can achieve this by performing switch operation using the WindowHandle method. In Selenium 4, this is going to change by using the new API newWindow.
So why do we need to create a new tab/window?
Let’s consider a test scenario where you have two interfaces, one for admin and another for users. As a user, you need to perform some steps then you have to log in as an admin. As an admin, if you do some configuration changes, these changes should be visible to the user. In such cases, we can open the website in one window and log in as a user. Then we can open the same website in another window and log in as an admin. Once the admin performs the configuration changes, we can go back to the user window and verify whether the changes are reflected.
How to handle the new tab/window in Selenium 4?
Selenium 3 vs Selenium 4
In Selenium 3, we needed to create a new WebDriver object and then switch to a new tab or window. But with Selenium 4, we can avoid creating new WebDriver objects and directly switch to the required window. We have a new API called newWindow in Selenium 4. This API creates a new tab/window and automatically switches to it.
New Tab
Here’s how you can create a new tab in Selenium 4 and switch to it:
Let’s consider that you are on the QED42 homepage and you need to switch to another URL in a new tab.
Here is the snippet to open an URL in the new tab:
New Window
Creating a new window in Selenium 4 and switching to it:
Similar to a new tab, here is the snippet to open an URL in the new window:
Switching back to the Original Window
Suppose, there are multiple tabs and windows open and you need to switch to a particular or original window/tab. This can be achieved using driver.switchTo().window(windowHandle) method.
Let’s implement the below steps to understand it:
- Load QED42 homepage using driver.get("https://www.qed42.com/")
- Open a new tab and load the other URL "https://www.google.com/"
- Next, open a new window and load "https://www.facebook.com/"
- Switch to the original window (step #1) by looping the windowhandles based on certain conditions and selecting the original window handle.
- Close the driver.
Below is the console output:
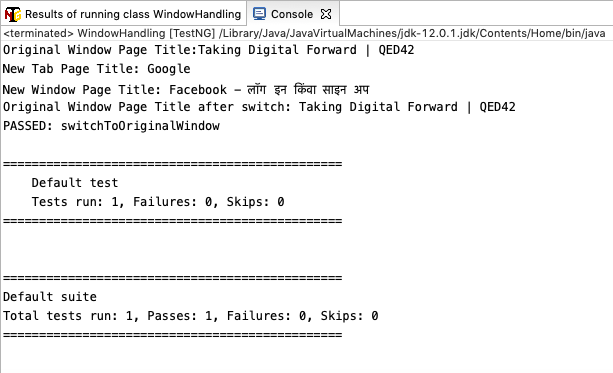
Point to note: Closing a window will leave WebDriver executing on the closed page and will trigger a No Such Window Exception. Therefore, you must switch back to a valid window handle in order to continue execution.
The sample codes are available at the Github repository here.
Conclusion
Selenium 4 provides better window/tab handling using a new API. We can now create a new tab/window and automatically switch to it with fewer lines of code and without creating a new WebDriver object.