New to JavaScript or need to brush up your concepts? This blog reviews the fundamentals of Functional Programming and Reactive Programming, the driving shift, what you need to know and which path is optimal for your requirements.
Functional Programming
Functional programming is a programming paradigm - Way of building a structure by composing functions to avoid change in State and mutable data.
Read more about why to use functional programming - http://bit.ly/2La6oVu
Features of Functional Programming:
- Functional programming is the process of building software by composing pure functions, avoiding shared state, mutable data, and it’s side-effects.
- They are declarative and not imperative.
What is Imperative and Declarative programming?
Imperative Programming: Type of programming which uses statements that change the current State of an application.
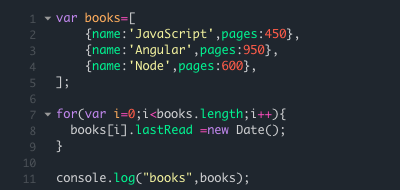

In this example, we have an array of objects with property names and it’s pages. Here we are using the for loop for iterating over an array, to add a new key - lastread with a date and display it on the console. Iterating this array using the for loop we are explicitly describing the logic to change the State.
Declarative Programming: Type of programming which expresses the logic of a computation without describing its control flow.
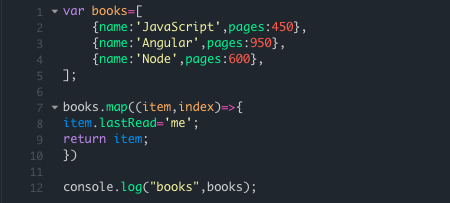

In this example, we have the same array of objects. Here we are using the map() function instead of for loop for iterating over the array. map() inbuilt iterates over an array and adds a new key which is displayed over the console. This program focuses on building the logic of software without actually describing its flow.
- Functional programming does not have any side effects because they are pure functions.
- Functional Programming distinguishes between pure and impure functions.
5 Concepts of Functional Programming:
- Pure Function
- Side Effects
- Shared State
- Immutable Data
- Function Composition
1. Pure Function - To understand what pure functions are let us compare them with impure functions. A pure function is a function which gives the same output for the same input arguments. Whereas an impure function is a function which gives a different output for the same input argument.


- sum() function has 2 parameters and it returns the addition of these parameters.
- It does not depend on any State, or data changed during a program’s execution. It only depends on its input arguments.
Impure function


- In the example, we have declared a variable - sum outside the function, and are adding that variable with the function's parameters.
- When we call the returnSum() function with the same argument it always returns a different output.
- In this impure function example, the variable sum is a side effect for that function.
2. Side Effect - Side effects may include I/O(input/output), modifying a mutable object, reassigning a variable, etc. Side effects are avoided in functional programming, which makes the effects of a program much easier to understand, and to test.
Functional programming does not have any side effects. It uses pure functions and pure functions are always side effect free. It does not depend on any State, or data change during a program’s execution. It only depends on its input arguments.
3. Shared State - What is a State? State is the attribute of a component at a given time.
Example - Consider, the strength of an office is 100 employees. At a given time there are only 50 employees present. So, the State of the office is 50 people.
Shared State is a State which is shared between two or more functions or components.
Example - A joint bank account shared between 2 people with the balance amount being 50,000/- rupees. This is a State. The 2 people are the components. If 1 person withdraws money from the bank, the updated amount will be reflected in the components. Therefore, the shared State is affecting the State of both components.
Thus, Functional programming avoids shared State.
4. Immutable data - Immutable means unchangeable.
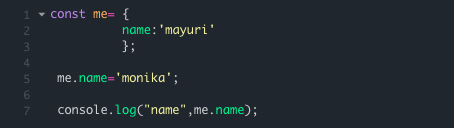

- We are using const in this example, const does not make our object immutable.
For making an object immutable we have to use an Object.Freeze() function. Object.freeze() is used to freeze objects.


A frozen object can no longer be changed. Properties can’t be added or deleted. So, the object becomes immutable. There are 2 methods for changing the properties of immutable data:
Object.assign() - In this function, we create a new object and clone the properties of the old object with changed properties/values.
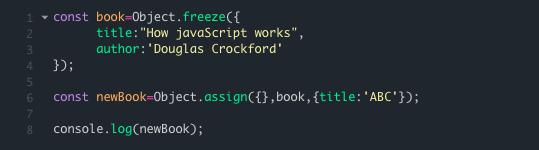

spread operator{...} - The 3 dots are the spread operator.

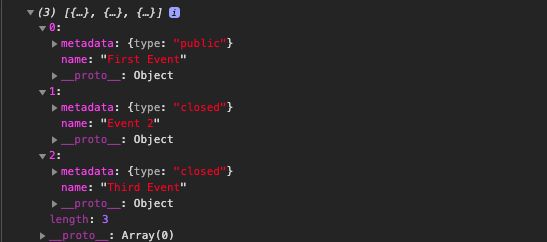
5. Function Composition - Function composition is a mathematical concept that allows us to combine two or more functions into a new function.
Example: f(g(x))
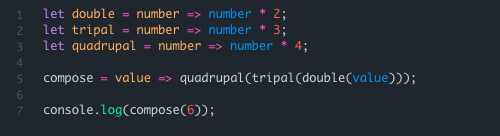

- Here we have four functions, the output of one function is used as an input for another function.
- When we call compose() function with parameter we get a cumulative output for all functions.
We use higher-order functions for the function composition. A higher-order function is a function that accepts other functions as an argument or returns a function as a return value. The best example of higher-order functions in JavaScript is that of Array.map(), Array.filter(), Array.reduce().
- map()- transforms an array by applying a function to all of its elements and returns a new array as an output.

- filter()- is used for filtering an array.

- reduce()- builds value by repeatedly taking a single element from the array and combining it with its current value. When adding numbers, it starts with zero and adds that to the sum.

Reactive Programming
Reactive programming is mainly concerned with asynchronous data streams.
Example: HTTP requests, or multiple repeatable actions (like keystrokes or cursor movements)
Observable, Observer, and Subscriber are the concepts of Reactive Programming.
Stream - A stream is a sequence of ongoing events ordered in time. It can be anything like variables, user inputs, properties, data structures, etc. It can emit three different things: a value (of some type), an error, or a 'completed' signal.
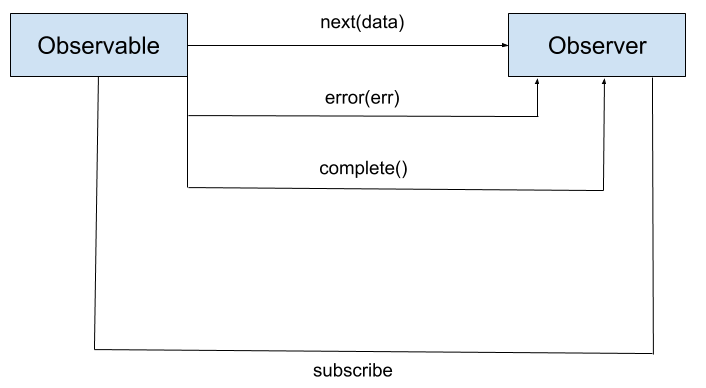
Observable, Observer, and Subscription:
- An observable is a function that produces a stream of values to an observer over time.
- When you subscribe to an observable, you are an observer.
- An observable can have multiple observers.
- An observable is a wrapper around a stream of values.
- They are lazy. They emit functions only when a value, error or complete signal is passed.
- They do not start producing data until we subscribe to it.
- An observer subscribes to the observable by calling the subscribe() method.
- Observables emit data and send it to the observer.
- Once again, observers read values coming from an observable.
- An observer is simply a set of callbacks that accept notifications coming from the observer, which include: next, error, and complete
Conclusion
One can use Functional programming or Reactive programming. It depends on the application you want to build and its requirements. Functional programming paradigm is built upon the idea that everything is a pure function. Reactive programming paradigm is built upon the idea that everything is a stream observer and observable philosophy.