Introduction
Now that you have set up your environment, it is essential to follow best practices when writing automation tests. These practices ensure maintainable, efficient, and reliable test code. This blog will discuss the best practices for writing Headway tests, including the Page Object Model (POM) design pattern and will also guide you through the basic structure of a Headway project, including the organization of pages, tests, and test data. It will also provide a sample code snippet of a Headway test class to search for a product on amazon.in.
Best Practices for Writing Headway Tests
The Page Object Model (POM) is a design pattern used in test automation to represent web pages as objects and organize them in a hierarchical manner. Each page is represented as a class that contains all the elements and actions that can be performed on that page. The POM pattern separates the page logic from the test code, making the automated tests more readable, maintainable, and reusable. The Headway framework employs the Page Object Model (POM) design pattern.
Benefits of using POM in organizing test code:
- Reusability: POM allows the same page object to be reused across multiple automation test cases, reducing the amount of redundant code and making tests more efficient.
- Maintainability: POM separates the page logic from the test code, making it easier to maintain and update test cases when changes are made to the page structure or functionality.
- Readability: POM provides a clear and organized structure for test code, making it more readable and understandable for developers and other stakeholders.
How to write maintainable and efficient automation tests using Headway:
- Use reusable functions: Create reusable functions for common actions such as clicking buttons, filling out forms, or navigating between pages. This will reduce the amount of duplicate code in your tests and make them more efficient.
- Use meaningful names: Use descriptive and meaningful names for your variables, functions, and test cases. This will make your code more readable and easier to understand.
- Follow a naming convention: Follow a consistent naming convention for your page objects and functions. This will make it easier to find and update them when necessary.
- Keep tests independent: Make sure that each test case is independent and does not rely on the results of previous tests. This will ensure that your tests are reliable and can be run in any order.
- Use assertions: Use assertions to verify that the expected results match the actual results. This will ensure that your tests are accurate and reliable.
- Use wait functions: Use wait functions to ensure that elements are loaded and ready before interacting with them. This will reduce the risk of errors and make your tests more robust.
Creating Your First Automation Test using Headway
Let's discuss the basic structure of the project:
Pages:
- The src/main/java/com/qed42/pages directory contains Java classes representing different pages of the web application being tested.
- Each page class represents a specific page and includes methods and locators related to that page.
Each page class typically includes:
- Element locators: These are defined using the By class from Selenium to locate web elements using different locators (e.g., ID, CSS selector, XPath).
- Action methods: These methods define the actions that can be performed on the page, such as clicking buttons, filling forms, or interacting with elements.
The headway-example repository project provides an example implementation of using Headway for test automation. For example, you'll find classes like ProductDetailsPage, CartPage, and SearchResultPage representing different pages of the web application.
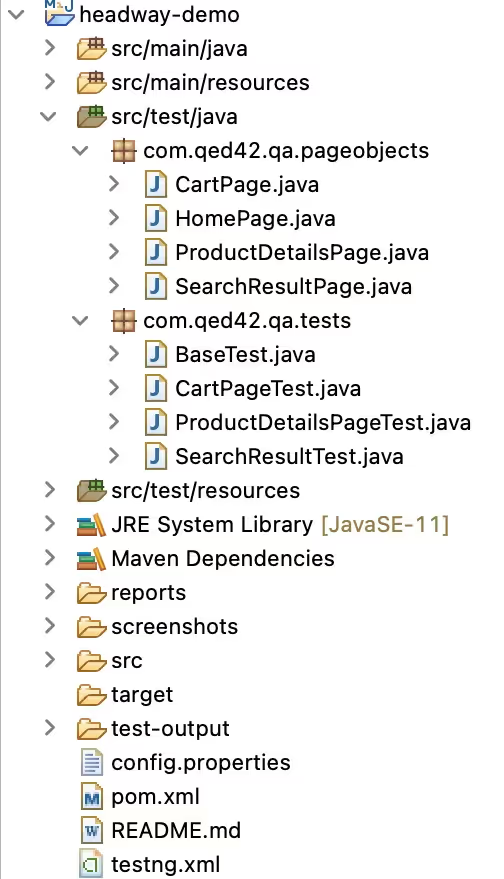
Below is sample code of SearchResultPage class that is used searching a product on amazon.in :
Tests:
- The src/test/java/com/qed42/tests directory contains Java classes representing the test scenarios for the web application.
- Each test class focuses on testing a specific feature or scenario and utilizes the page classes and their methods.
- The BaseTest class uses TestNG framework annotations to define test methods and setup/teardown methods.
- Test methods are typically annotated with @Test and use the page classes to interact with the web application and perform assertions.
- For example, you'll find classes like CartPageTest, ProductDetailsPageTest and SearchResultTest containing test methods that verify the behavior of the cart and search for the product.
Test Data:
- The src/test/resources directory contains test data files or resources that can be used in the tests, such as sample data in csv or json or test configurations.
Below is sample code of SearchResultTest class that is used searching a product on amazon.in :
You can refer to Chapter 3 of Headway user guide to get more information on how we have automated a few use cases for amazon.in.
Debugging Your Headway Test
Debugging tests is an important part of test automation. Here are some tips and tricks for debugging your Headway tests:
- Add log statements: Insert log statements at critical points in your test code to output relevant information, such as the values of variables, the execution flow, or error messages. Log statements can be printed to the console or written to log files, depending on your logging configuration.
- Use test reports and screenshots: Headway framework generates test reports and captures screenshots during test execution, you can review them to identify any unexpected behavior or errors. These reports can provide valuable insights into the state of your tests and the application under test.
- Inspect element properties: During test execution, you can inspect the properties and attributes of web elements using debugging tools such as browser developer tools. This helps ensure that the elements are correctly located and interacted with in your test code.
- Use breakpoints: Set breakpoints in your automation test code to pause the execution at specific points and examine the state of variables and objects. This allows you to step through the code and identify any issues or unexpected behavior. IDEs like IntelliJ IDEA, Eclipse, or Visual Studio Code provide convenient ways to set breakpoints.
Conclusion
Congratulations on creating your first Headway test! By understanding the structure of a Headway project and utilizing page classes, test classes, and test data, you can create effective tests to automate web application testing. With Headway, you can confidently verify the behavior of web applications and ensure their quality. By following the tips and tricks discussed in this blog, you can enhance your debugging process and ensure the reliability of your tests.