A simpler way to animated SVGs in React Native.
Animations are an important feature that one can include in his/her app. They give a much better user experience and when it comes to SVGs, animations become all the more important. But as you may already know, they are not supported in React Native by default. Which means if you take an online animated SVG and use it in your React Native app, you will not see its animations as you would see on a browser.
This blog is an attempt to work around this shortcoming of React Native. There are a few online libraries available for the same, but none are well explained and there are not many blogs about this. So, sit tight, as we discuss the implementation of animated SVGs via an example.
Prerequisites
- We will be needing some of the properties of the SVG that we need to animate. These can be obtained by opening the SVG file in any code editor or in any SVG to JSX converter available online.
- We will be using an online library react-native-svg-animations with some modifications and hence it should be installed beforehand.
Getting Started
We will choose an animated SVGs from loading.io and understand how its animations will be implemented in React Native. The SVG that we will be implementing is:
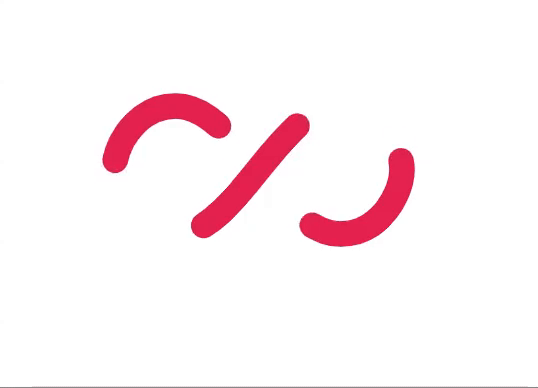
Let’s see what properties we will be using. Following is the code obtained as we convert SVG into JSX:
The most important property that is needed is the d prop that is passed on to the <path> component which defines the path to be drawn. It is a list of path commands in the form of letters and numbers.
Apart from this, we will be using the strokeDasharray property for our example which is used to create dashes within a stroke. So, instead of a single stroke completing the animation, there will be multiple strokes with dashes / blank spaces between them. The number and length of strokes are defined by the length and the values of the array that we pass to this property.
Implementation
We need to import AnimatedSVGPath from the module that we installed.
We will be implementing the following render method:
Now if you look at the properties of this component, you will notice that it uses the d and strokeDashArray properties that we obtained earlier from the SVG.
You can read and understand more about this module here
Apart from this, we added an onPress event on <TouchableOpacity> to check whether it hinders the animation of SVG in any way.
Modifications to the Installed Module
While using react-native-svg-animations we faced the following issues :
- ISSUE WITH STROKEDASHARRAY PROPERTY
strokeDashArray was not a part of its props originally, so we needed to modify the original module in order to enable users to pass values on their own. Consider the return method of the AnimatedSVGPath class:
If you look at the strokeDasharray property of the <Path> component, its value was originally [ this.length, this.length ] and the user was not able to define the number of strokes he/she needs in the animation. We modified it by adding a condition { dashArray || [ this.length, this.length ] } to enable users to pass its value through props according to their requirements.
Before and after using strokeDasharray property:
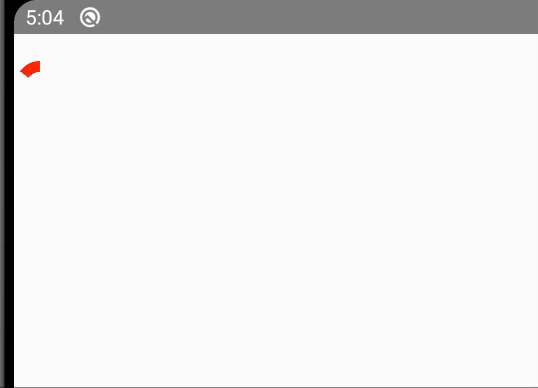
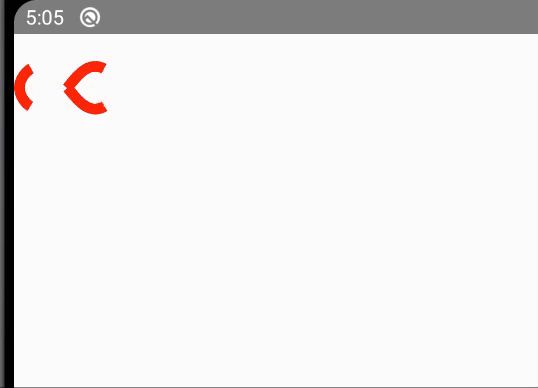
- DELAY ISSUE BETWEEN EACH ITERATION
After solving the first issue and successful implementation of the animations, we experienced a little delay between each iteration of the animation. This can also be seen pretty clearly in the above gif. After some research, we found out that we need to add easing field to the Animated.timing function inside the animate method as shown below:
Easing needs to be imported from ‘react-native’ first and then by providing easing: Easing.linear, the delay between each iteration is eliminated.
Before and after using Easing property:
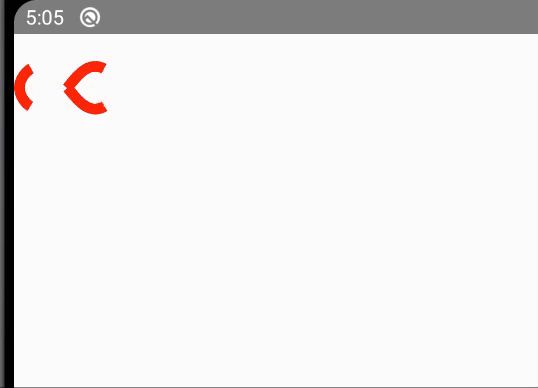

It is clearly visible from the above gifs that the issue has been fixed.
So, these were the two issues that we faced while working with this module. We fixed them and also raised a PR for the same. There won’t be an issue if you intend to use the same library in future.
Wrapping this up!
With the issues fixed and everything explained, now anyone can implement SVG animations in React Native without any hassle. You can check out the example code here in case you wish to have a look at the whole code. This will give you more insight into what we did here.
It was really fun to work on this, learnt a lot and tried out some cool things with SVG animations. I hope this blog sails you through if you ever find yourself in the middle of this ocean!
Keep learning!