As per the React documentation "Context provides a way to pass data through the component tree without having to pass props down manually at every level.” But, without diving straight into the definition of what Context API is, let’s try to visualize our problem first.
Prerequisite
Basic understanding of stateful functional components in React.
Consider the example explained in the chart below.
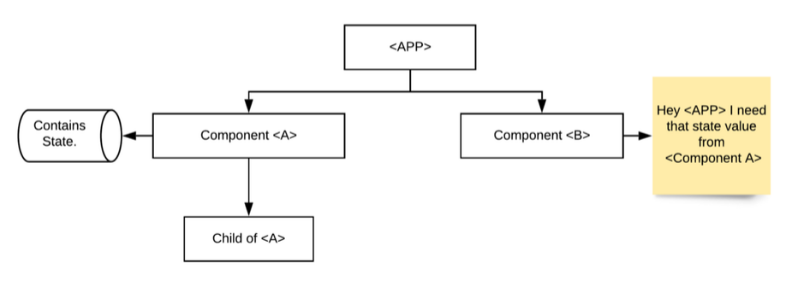
- Both Component A and B are rendered in the main APP component.
- Component B requires some state value from Component A
- This is not possible, right? Because all the state values reside in Component B
The solution to this problem can be uplifting the state to your Parent APP component. Which will look something like this:

But this is not the ideal solution because as your APP grows larger and larger you can’t keep the state values in your Parent Component. So, what is the solution?
This is where the Context API comes into the picture

- Context API is the way to create global variables that can be passed around the component tree.
- Context API is an alternative to passing props manually in a component tree. Something that is also called Prop drilling.
- And as per the React documentation: Context is primarily used when some data needs to be accessible by many components at different nesting levels.
Let’s create our Context API
First and foremost, let’s set up our directory structure. Take any basic example that follows the above-mentioned problem.
Our directory structure will look something like the above flowchart.
Your Parent APP component should look something like this:
Step1: Create a Context.js file anywhere in your Component folder.
Add the below piece of code to your file. This will create a context named AppContext
Step 2: Next let’s create our Provider. The Provider is where we provide all our values to the components consuming the context.
Step 3: Wrap your Parent Component with the Provider:
Step 4: Now you can consume the context value anywhere down the AppProvider Component tree. By using the useContext hook:
Things to Remember while using Context
- Context should not be used as a replacement for the state, to avoid prop drilling. Always use the local state over the global state when you can.
- If you want to change something in the context (say if we change the message in the above code by using the SetMessage method), every time you update that state value it will re-render all the components that use the Context.
- Don’t wrap your whole App inside the Context Provider, this can be an overkill. Try to narrow down the component tree to the lowest possible Parent component that can be wrapped within the Provider.
Conclusion
- Use createContext() to create the Context
- Pull the Provider out of Context created from createContext()
- Wrap your Parent component with the Provider
- Consume the context with useContext() hooks
If you are from the redux world and haven't heard of Context API before. Visit /insights/coe/javascript/reacts-context-api-and-how-it-can-replace-redux to know more about Context API and how it can be a replacement over redux for small scale applications.
Hope this blog post was informative. Do send us your queries in the comment section below.