In this article, I will show you how to expose data from a custom table with the help of RESTful services and JSON. Such cases usually arise when you need to expose data for other sites or want mobile development for an existing Drupal site.
To do this we need Drupal 7 and services module. In addition, you will also need to create one custom table by writing code in .install file (I hope you know what I am talking about).
For this purpose, you may use drush for downloading "Services" module. Using Drush, you could type the following in terminal - drush dl services and then type drush en -y services to enable to it.
After enabling these two modules, follow the steps as mentioned below:-
1. Add a service in services UI
Go to service UI page(admin/structure/services). Here, you need to enter endpoint and choose the server.
Click on the add button and after that the following picture will be displayed.
There you need to put machine readable name, select the server "REST" and define endpoint, here I put "setindia_api".
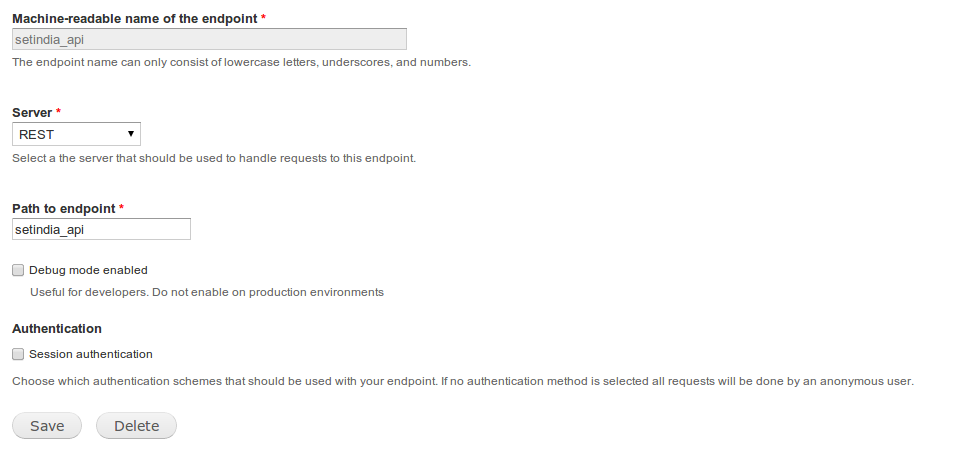
For testing purpose enable Debug mode. And then click on save to complete the process.
2. Open Resource page
This page will be displayed in the service list. From there you can click on edit resources.
You should see the following screen:-

Resources are content available for query through the end points. As you look at the default setup, you see those resources, that come hard-coded with the Services module. But our resources is not showing there yet. We will need to create one custom module by which we will able to do so.
3. Create a Custom Module
If you don't know to building custom module, please refer this linkhttp://drupal.org/node/1074360, there you will find everything. Here I will only cover which is needed for build custom web services with the help of RESTful services.
For our convenience, I will call the module CUSTOM_MODULE.
4. Declare a Service Resources
There is hook provided by Services module i.e hook_services_resources().
Following is the code which we will require:-
In the code above, we declare a Resource called country_field_values, with a function "retrieve". We allow one optional parameter, "nid". The data will be accessible for a user with access content permission, and the params will be passed to a callback function _MYMODULE_country_retrieve().
5. Create the callback function()
Here is the callback function:-
Here I fetched the argument and passed it to the other function, calling it in the process. One need not call another function during return, but I do so, such that I can optimise the params I am passing to it.
6. Create the Processing function()
Here the is required code:-
Here we are fetching all the required component and return back.
We are almost done, so hang on.
7. Enable the resource
Enable the require resources, in our case we are enabling country_field_values.

After enabling the resources, type in url like this $base_url/setindia_api/country_field_values/[country_id]?nid=[nid].
Here [country_id] could be the variable which we are passing in $fn and [nid] is nid.
After hitting the above url, this will return like this:-
Here,country_id I was passing "4" and nid was "9194".
This is showing like this because we are only allowing JSON.