Managing and importing podcast feeds into your Drupal website can greatly enhance your content diversity and keep your audience engaged. In this guide, we'll walk through the process of setting up a podcast feed integration using Drupal, covering important aspects such as parsing feed data, creating a custom parser, and saving relevant information as media entities.
Prerequisites
Before diving into the integration process, ensure you have the following elements in place:
- Drupal website (preferably Drupal 8 or Drupal 9)
- Feeds module installed and enabled
- Custom module for extending Feeds functionality
Setting Up the Podcast Feed Parser
1. Create a Feed Parser
Inside your custom module directory, create a Feeds/Parser subdirectory. Within this directory, develop a custom parser class to handle the podcast feed. This class should extend the Feeds\Parser\ParserBase class.
// CustomModule/src/Feeds/Parser/CustomPodcastParser.php
2. Implement Parsing Logic
Inside the custom parser, implement the parse() method to extract relevant data, such as podcast duration, from the feed. Additionally, use the getMappingSources() method to define the mapping source for the new podcast duration field.
// CustomModule/src/Feeds/Parser/CustomPodcastParser.php
Creating the Event Subscriber
3. Event Subscriber for Presaving
Create an event subscriber within your custom module to handle presaving of entities. This subscriber should fetch relevant information, such as the podcast thumbnail and audio URL, and save it as a media entity.
// CustomModule/src/EventSubscriber/CustomPodcastEventSubscriber.php
4. Configure Event Subscriber
Finally, configure the event subscriber to trigger the presave function when necessary. You can do this by creating a services YAML file.
# CustomModule.services.yml
Example Feed Data
Here's an example of podcast feed data for reference:
Steps to configure Feed on Drupal UI with custom parser.
Step 1: Navigate to the Feeds Page
To begin the configuration process, access the Feeds page on your Drupal site. This can be achieved by navigating to /admin/structure/feeds.

Step 2: Add a New Feed Type
Once on the Feeds page, click on "Add Feed type" to initiate the setup process for a new feed.
Step 3: Provide Feed Details
Enter the necessary details for the feed you're configuring. For example:
Name: Podcast
Fetcher: Download from URL
Parser: Custom Podcast
Processor: Node
Content Type: Podcast
These details ensure that Drupal knows how to handle the incoming feed data.
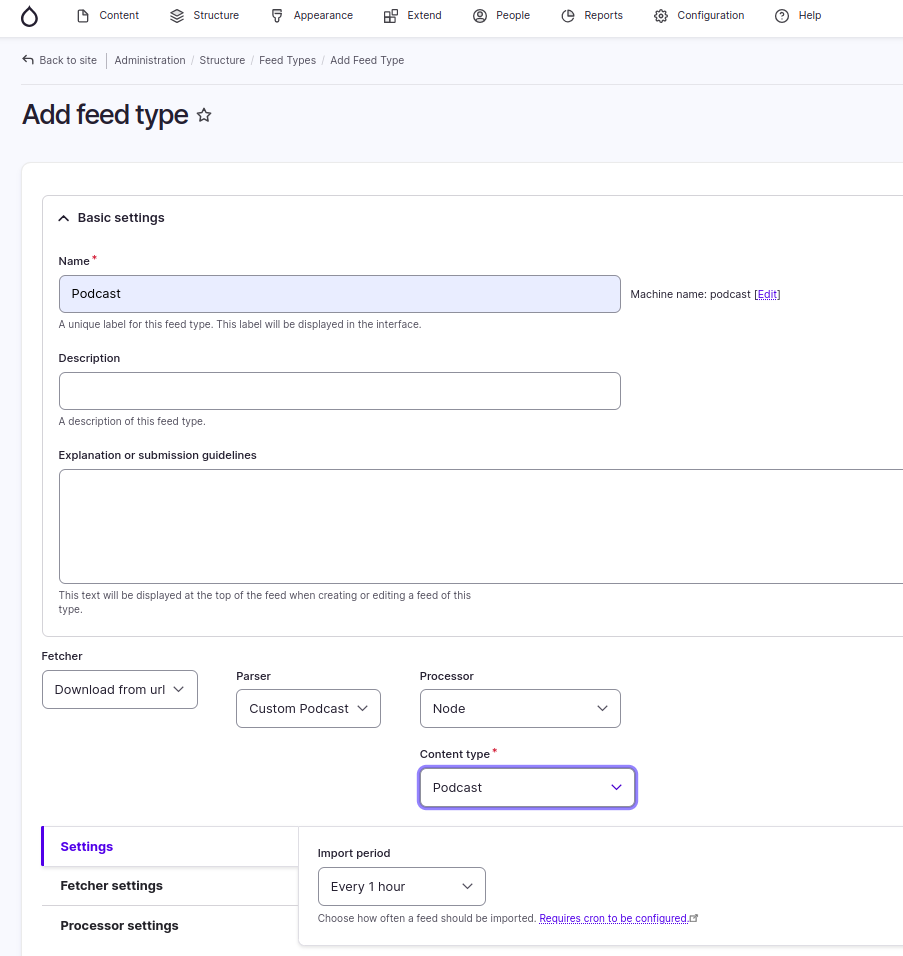
Step 4: Save the Feed Configuration
After entering the feed details, save the feed configuration to proceed to the next steps.
Step 5: Configure Field Mapping
Navigate to the Mapping tab, where you can map the fields from your feed to corresponding Drupal fields. Here, you'll find the Duration field in the source field list. Configure the source and target fields according to your feed data, and then save the mapping settings.

Step 6: Prepare for Feed Import
With the field mapping completed, your feed is now ready for import. You can proceed to the Feeds section under the Content tab (/admin/content/feed) and add a new feed.
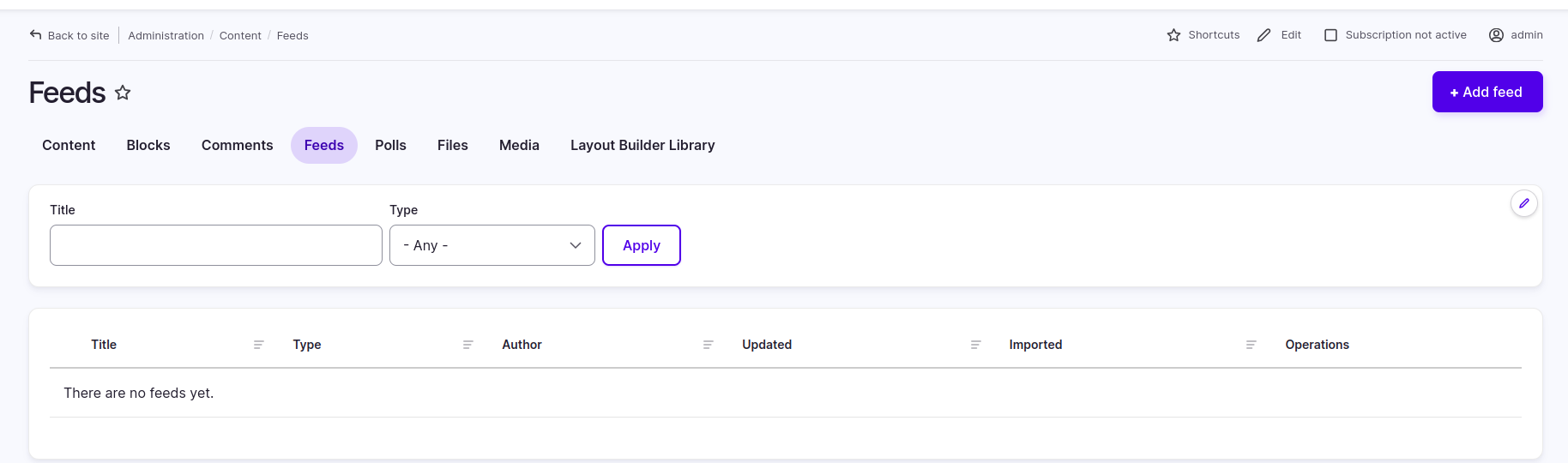
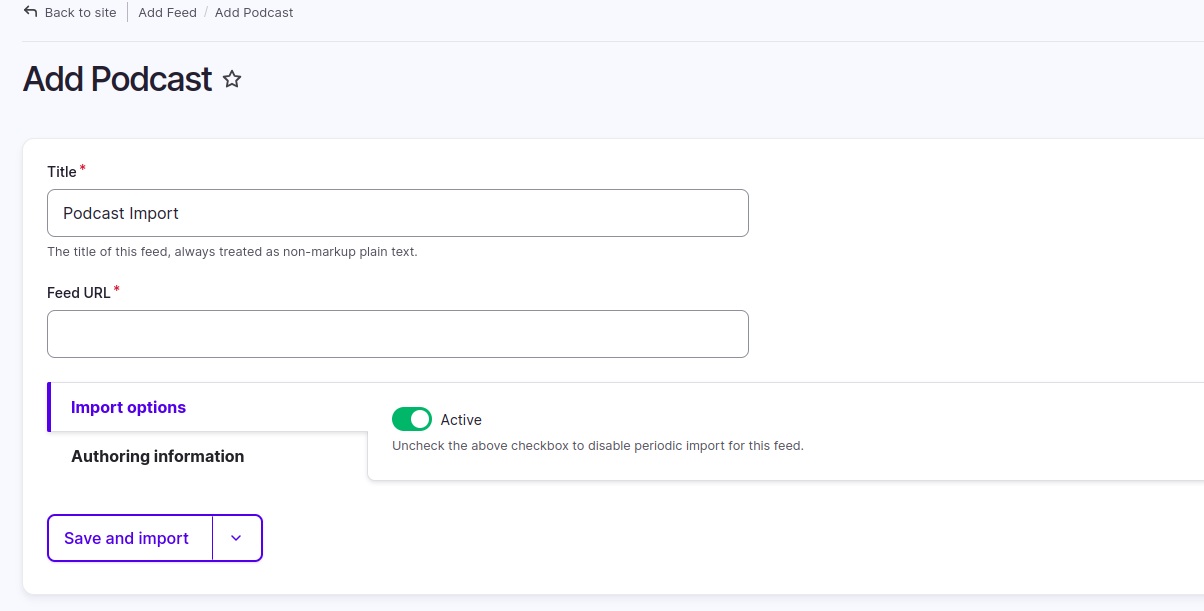
Step 7: Import and Verify
Initiate the import process for the added feed, and verify the imported data in the content list. This step ensures that the configured feed is successfully pulling in content according to your specifications.

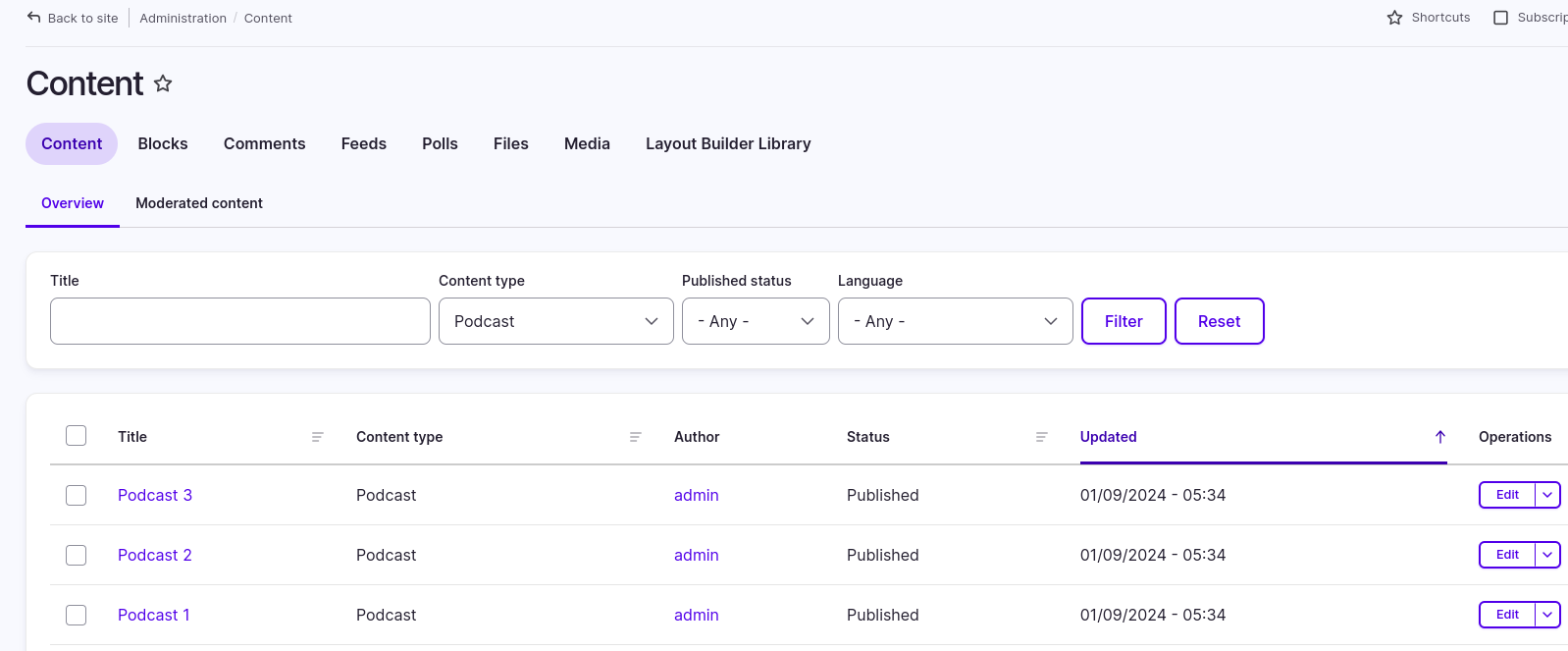
Conclusion
By following these steps, you'll have successfully set up a podcast feed integration in Drupal. This not only allows for seamless content updates but also enhances the overall user experience on your website. Adjust the provided examples according to your specific requirements and feed structures.
Happy podcasting!