What is State? And why do you need to manage it?
The State is an important concept in React that stores data and displays the behavior of a component. People choose different approaches for managing State in React. Let us delve into the basics of React State and different ways to manage it.
Updating the State of a component is easy. But what if you want to update it in child component? This is where it becomes tricky.
In React, State is immutable. It should not be altered directly. For updating the child component, you need to pass a function to the child as props, which will then update a parent State. It cannot be mutated directly in the child. This approach is efficient if the scope of the project is smaller. Whereas, in large project component structure (a project heavy on hierarchy), it would be a repetitive job which makes no sense.
The ultimate objective is to improve the performance of an application. As a React user, your objective is to minimize the use of State and it’s mutation.
State management can be omitted. One can build an application in a much better manner without using State management too. Here are the scenarios where people may need to manage application State:
- Updating parent State
- Maintaining the code structure of a complex project(Redux)
- Dynamic updates of components (Observables)
- Increasing performance and reduce redundant work
- Storing variables in one place(Store) enables easy access and code quality is maintained. It also makes it easy for other developers to understand project structure
- Easy Dom rendering
Tools of State management
1.Services
What is a Service?
Service is nothing but a set of functions at one place. You can store variables and functions that are needed by more than one component in the Service. Service can be accessed directly by importing it in the required components, or by providing it to a parent and passing as props to other children.
Example: This is one of the simple solutions that we have tried with to manage State instead of having other complex State management tools.
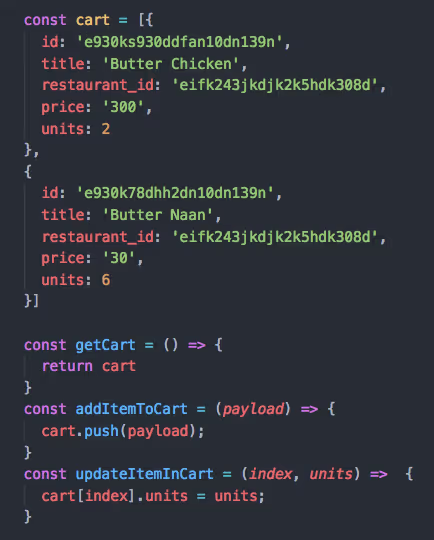
Above example contains a service consisting JSON array of an e-commerce cart with data and functions to access or update the cart.
Using Service is a good approach to maintain the component State but it also restricts the use of other React features like observables.
2.Redux
Redux is a design pattern used to structure your application. It maintains a single immutable Store and a function that returns a new State on every action.
Redux structure:
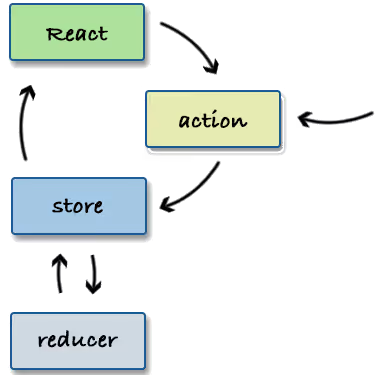
The three concepts used in Redux are:
- The Store is simple ES6 class where variables are placed.
- Actions are the user events that occur and are passed to the Reducer function as an argument.
- Reducer is a simple function which takes two input parameters as; ‘store’ and ‘type of action performed’ and mutate Store values.
The interesting part of Redux is that the changes will only affect the initiated component and respected variables from the Store. Applications without using Redux need to change everything in the component hierarchy. On the other hand, Redux mutates the Store values and reflects those variables of the Store directly in the components which are using those. It will not check the hierarchical function of components to change the State of that particular component. To learn more about Redux please refer - https://redux.js.org/introduction/getting-started
When to use Redux?
- Multiple props need to be passed to many components
- Structured code is required
- Need to store global variables in one place
- Large team looking for a maintainable code
- For complex applications
When not to use Redux?
- Projects of higher magnitudes should not use Redux since it is difficult to manage. Redux makes it more complex to handle and to understand.
- For every change made to the Store, one has to access updated Store values on UI.
3.Mobx
Mobx has two parts:
- Stores: Unlike Redux, Mobx can have multiple Stores in a project
- Observables: Store exposes observable fields and an observer which react upon changes made to the Store

Why Mobx?
- Since data is observable, the observer will react to it automatically
- No need to manually access Store variables for the UI components
- Increases performance
- More data abstraction
When to use Mobx?
- For small and simple applications
Mobx is much easier to learn because the majority of JavaScript developers are familiar with OOP. There is a lot of abstraction in Mobx and is easy to test. To learn more about Mobx refer - https://medium.com/react-native-training/ditching-setstate-for-mobx-766c165e4578
4. Context API
Context API is used to share data between components without explicitly passing props to every level of the component tree. It is suggested to use props if a project has few levels of children.
Concepts used in Context API:
- Context Object
- Context Provider
- Context Consumer
5.Hooks
As an application grows managing Stateful components become difficult. Hooks allow us to break components into smaller functions. You can also write a custom hook. To learn more about hooks please refer - https://reactjs.org/docs/hooks-overvie
Conclusion
Complex applications lead to complex State management. Cases where multiple components need to be handled and could increase in time, you may choose any of the React State management tools. Since a simple and easy application is always desired, avoiding State management would be advised. However if in case a project needs to have component State then it needs to be handled properly. The more you care about State management the more complexities arise.
Credits
Abhay Kumar.