React (a JavaScript library) has been around in the market for around a decade and it is the first choice for most of the developers. React is performant but at times one would like to optimize their apps as the app size grows.
If you are using the create-react-app tool to get started with your react apps then this article will surely interest you. Most of the methods for optimizations generally deal with changing webpack’s configurations. You will not have access to these configurations until you eject your app using ‘npm run eject’, after which you have to directly configure the webpack.
Let us explore 2 easy steps of optimization:
- Tree Shaking
- Code Splitting
In order to optimize we need a current representation of our app which will help us in executing the required steps.
Prerequisites
1. Add ‘source-map-explorer’ as a dev dependency.
2. Edit your package.json file and add “analyze”: “source-map-explorer ‘build/static/js/*.js’” to your scripts property.
3. Run command 'yarn build'.
4. Then run 'yarn analyze'. This may take a while, depending upon your app size.
5. Once the analysis is complete, a new tab will open in your browser, where the production bundle is displayed.

Step 1: Tree-Shaking
The above image is a representation of how much size is occupied by each library. We won’t be using all the functionalities of libraries having bigger footprints. Thus, it's better to exclude unwanted components and libraries. In my case, it’s material-ui a UI library from which only selected components were used. Instead of importing the whole library and extracting components using destructuring, you can directly import the components from their libraries by simply replacing:
with
Once the above steps are performed for all the components that were imported improperly. Build your App again and run ‘yarn analyze’.
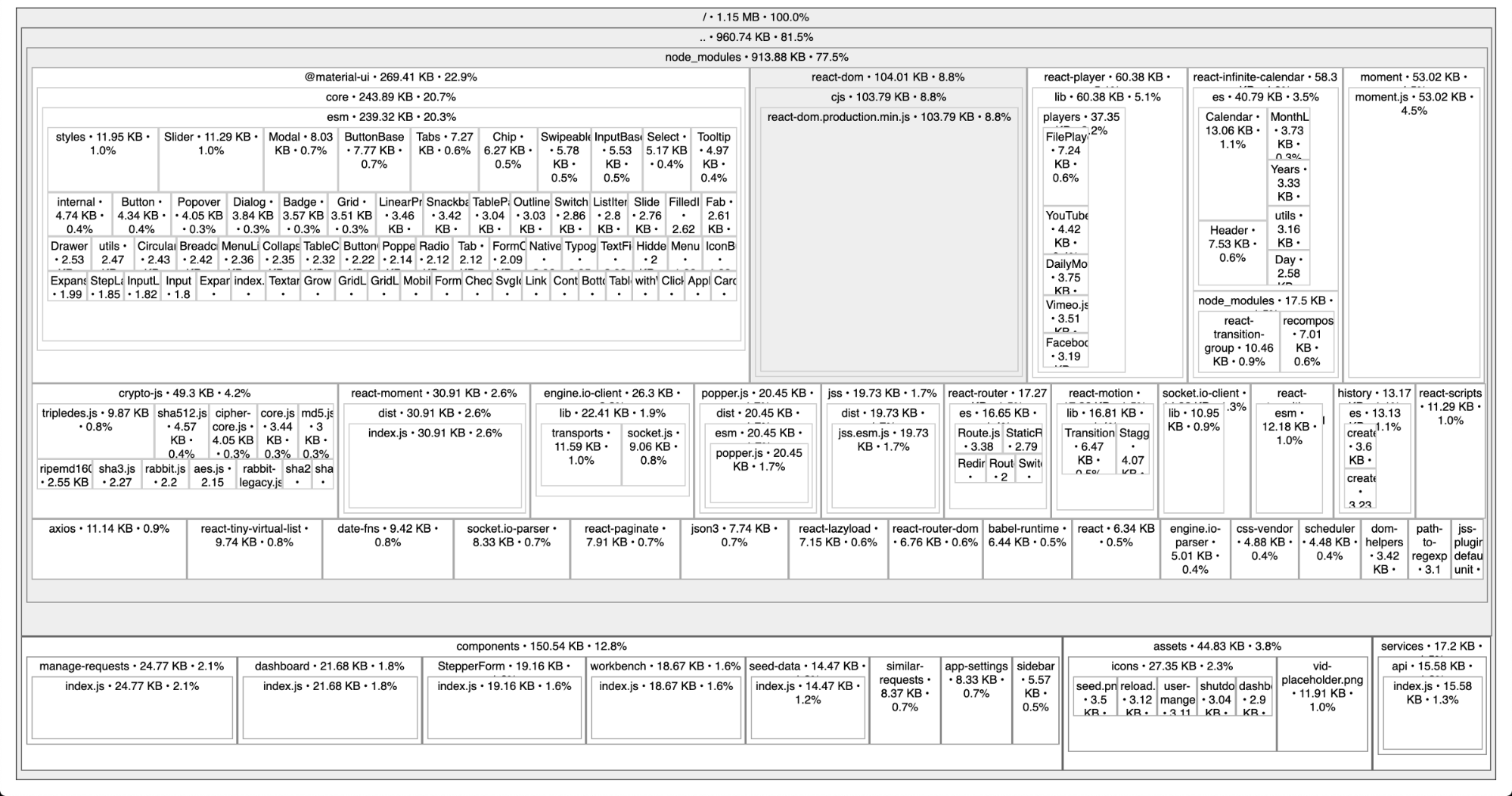
From 269KB down to 184KB, pretty impressive right?
After getting rid of all the unused imports and only having used components in our build, the next step that makes sense would be to chunk certain parts of our app bundle that are not critical for the first load.
Step 2: Code Splitting
We will split our app’s main bundle using React.lazy and React.Suspense which enables us to lazily load our components.
In this case, react-infinite-calendar is a module for filtering some data and is not frequently used. So, it's better to load it when required.
- Import Lazy and Suspense in your component.
- Lazy load your component.
- Wrap your component around Suspense and don’t forget to provide a fallback component.
Note: You will have to lazy load all the instances of the component you have chosen. In my case, there were 3 files where I was using react-infinite-calendar.
After this, your component will be available as a different chunk file which will only load when required. Look at the network inspector.
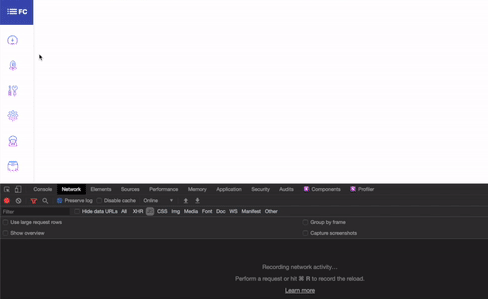
There are different ways of optimizing an App, and they can vary depending on the architecture, use-cases, and a lot of other things. The above-mentioned steps are the simplest and applicable for any React App.